Writing to the Event Log
Event logging is the centralized way for Windows to record information about important software and hardware events that occur across a system. For System Administrators the logs allow them to review errors. For developers the event logs provide a place to store application errors. Within the .NET Framework the System.Disagnostics.EventLog namespace provides the functionality to read, write and manage events logs and events on the network. In this post we will look at some examples of how this namespace can be used within your applications.
Reviewing the Event Logs
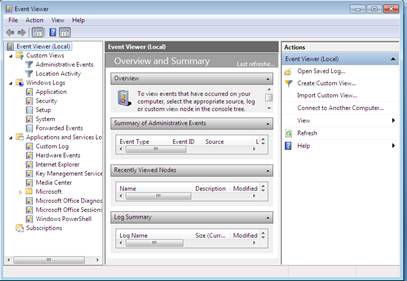
The Event Viewer shown above is the Microsoft Management Console (MMC) snap-in that enables a system user to browse and manage the event logs. This is the main interface for reviewing event logs.
Note: When using the Event Viewer to review logs you will want to log in as administrator. Otherwise you can only change settings that apply to your user accounts, and many logs may not be available.
Starting with Windows Vista the event log hierarchy was simplified down to Windows Logs and Application and Services Logs. Windows logs are intended to store events from applications and events that apply to the entire system. The Windows Logs category includes the event logs that were available on previous version of Windows. This includes the Applications, Security and System logs. The Setup log and Forwarded Events logs are new.
Applications and Services logs are a new event log category. These logs store events from a single application or component rather than events which may have a system wide impact. This category of logs includes four subtypes as shown below.
Log Type
|
Description
|
Admin
|
These events are primarily targeted at end users, administrators, and support personnel. The events that are found in the Admin channels indicate a problem and a well-defined solution that an administrator can act on. These events are either well documented or have a message associated with them that gives the reader direct instructions of what must be done to rectify the problem.
|
Operational
|
Operational events are used for analyzing and diagnosing problems.
|
Analytic
|
Analytic events describe program operations and may indicate problems that cannot be handled by direct user intervention.
|
Debug
|
Debug events are used by developers to troubleshoot issues with their programs.
|
Rather than having to work between Visual Studio and the Event Viewer during development. Visual Studio provides the Server Explorer which contains the same basic information as the Event Viewer. The Server Explorer shown below is available from the View Server Explorer menu.
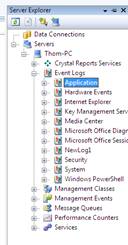
Implementing the EventLog Class
. Within the .NET Framework the System.Diagnostic namespace contains the EventLog and EventLogEntry class that allows .NET developers to interact with the event logs. The EventLog class allows .NET developers to read, write, delete and create new event logs. The EventLogEntry class encapsulates a single record in the Event Log.
Listing the Event Logs
As an example to find the existing logs on a computer we can use the EventLog.GetEventLogs method that searches all the event logs on the local computer and creates and array of EventLog objects. The following code example retrieves the list of event logs on the local computer and then displays the name into a textbox.
private void bListLogs_Click(object sender, EventArgs e)
{
EventLog[] localEventLogs;
localEventLogs = EventLog.GetEventLogs();
textBox1.Text = "Number of logs on local computer: " + localEventLogs.Length + "\r\n";
foreach (EventLog log in localEventLogs)
{
textBox1.Text += "Log: " + log.Log + "\r\n";
}
}
When run this returns the following into a tecbox.
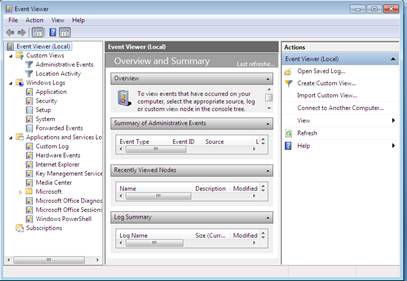
Validating the Data Source
In order to write to an event log the application must specify an event source. The event source indicates what logs the event. Typically a source may be named after the application or component that is creating the event entry. When an event entry is written the system uses the source property to find the appropriate log in which to place the entry.
In order to create or write to an event source you need to have administrative rights on the computer. By default all event logs, including security are searched to determine whether the event source is unique. In Windows Vista and Windows 7, User Account Control (UAC) determines the privileges of the user. If you are a member of the Built in Administrators group, you are assigned two run time tokens; a standard access token and administrator token. By default you are in the standard user role. To execute code that accesses the security log, you must first elevate your privileges from a standard user to administrator.
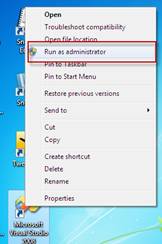
As shown above, this can be done by right clicking the application and indicating that you want to run as administrator.
When the source is created it registers the application as a valid source of entries to the log. Each source can only be used to write to one log at a time. However, a single event log can be associated with multiple sources. Even though the source property is a string, it must be unique from any other sources on the computer. If the source for the event log does not exist a new event source is created. For example the following code example placed with a button control will validate that a source exists.
private void bSourceExists_Click(object sender, EventArgs e)
{
bool sourceExists = System.Diagnostics.EventLog.SourceExists("KenticoSource");
MessageBox.Show("is the source there - " + sourceExists.ToString());
}
Reading from the Event Logs
The follow example will read from the Application Event Log on your local machine and print out the time the errors were generated into a textbox.
private void bReadLog_Click(object sender, EventArgs e)
{
EventLog theLog = new EventLog();
theLog.Log = "Application";
foreach(EventLogEntry myEntry in theLog.Entries)
{
textBox1.Text+=" Time Generated:" + myEntry.TimeGenerated.ToString() + "\r\n";
// Examples of other things that can be added
//textBox1.Text += "Source:" + myEntry.Source;
//textBox1.Text += " Message:" + myEntry.Message;
}
Creating a Custom Log
The following example will create a custom event log called KenticoLog.
private void bCustomSource_Click(object sender, EventArgs e)
{
EventLog eventLog1 = new EventLog();
// Source cannot already exist before creating the log.
if (System.Diagnostics.EventLog.SourceExists("KenticoSource"))
{
System.Diagnostics.EventLog.DeleteEventSource("KenticoSource");
}
// Logs and Sources are created as a pair.
System.Diagnostics.EventLog.CreateEventSource("KenticoSource", "KenticoLog");
// Associate the EventLog component with the new log.
eventLog1.Log = "KenticoLog";
eventLog1.Source = "KenticoSource";
}
If you refresh the server explorer you will see that the new event log is available.
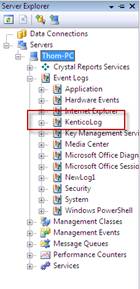
Writing to an Event Log
The WriteEvent and WriteEntry methods are used to write events to the event log. When writing events, at the very least you must specify a message string or the resource identifier for the message string. M any of the other properties are optional. As an example, the following code will write an error message to the KenticoLog we created earlier.
private void bWriteEntry_Click(object sender, EventArgs e)
{
EventLog theLog = new EventLog();
theLog.Log = "KenticoLog";
theLog.Source = "KenticoSource";
theLog.WriteEntry("This is an informational message");
theLog.WriteEntry("This is an error message", System.Diagnostics.EventLogEntryType.Error);
}
Once the code is executed as shown below you can review the new entries in the event log within the Server Explorer.
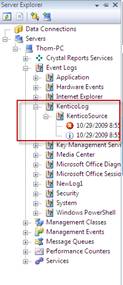
It is important to remember that Event logging consumes disk space, processor time and other system resource. It is important to log only essential information. A good rule of thumb is to place event log calls in an error path of your application, rather than the main code path so that you dont adversely affect performance of your application.
Download the code example used in this project from here