Automating Twitter for New Blog Posts
Like most people once I finish a blog post, the next step is to send out a tweet. Seemed like a lot of work and something that should be automated. In this post I will show how you can automate the process of sending a tweet whenever a new blog post is added. Next, I am planning on cleaning the code up a bit and wrapping it within a module. If youre interested in helping with the sample let me know. I am thinking if we get enough people interested we can create a CodePlex project.
Twitter enables access using a REST based API. The API is fairly easy to us. In order to send a twitter from .NET you can use the following code.
/// <summary>
/// Post an update to a Twitter acount
/// </summary>
/// <param name="username">The username of the account</param>
/// <param name="password">The password of the account</param>
/// <param name="tweet">The status to post</param>
public static void PostTweet(string username, string password, string tweet)
{
try {
// encode the username/password
string user = Convert.ToBase64String(System.Text.Encoding.UTF8.GetBytes(username + ":" + password));
// determine what we want to upload as a status
byte[] bytes = System.Text.Encoding.ASCII.GetBytes("status=" + tweet);
// connect with the update page
HttpWebRequest request = (HttpWebRequest)WebRequest.Create("http://twitter.com/statuses/update.xml");
// set the method to POST
request.Method="POST";
// set the authorization levels
request.Headers.Add("Authorization", "Basic " + user);
request.ContentType="application/x-www-form-urlencoded";
// set the length of the content
request.ContentLength = bytes.Length;
// set up the stream
Stream reqStream = request.GetRequestStream();
// write to the stream
reqStream.Write(bytes, 0, bytes.Length);
// close the stream
reqStream.Close();
} catch (Exception ex) {/* DO NOTHING */}
}
The good news is that twitter contains a variety of other methods and I can easily see ways to take advantage of other areas. I did some searching and found there are a lot of free .NET .NET libraries available to simplify the coding process. After some search I ran across the Yedda Twitter API. It was pretty easy to use and really reduced the amount of code needed. (If you have any reviews of Yedda or another library would be very interested in hearing them)
Once I added the Global Event project and the Yedda Library to my code my Solution Explorer looked like the following.
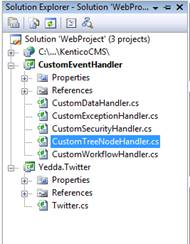
Given the character limitation on a tweet, shortening URLs is important I used the bit.ly API for this. For more information about this take a look here.
Onto the code for the Global Event when a new blog post is made the Global Event captures the insertion and fires the URL shortening and the posts the tweet.
public override void OnAfterInsert(object treeNodeObj, int parentNodeId, object tree)
{
// INSERT YOUR CUSTOM AFTER-INSERT CODE
// type the document as TreeNode
TreeNode newsDoc = (TreeNode)treeNodeObj;
switch (newsDoc.NodeClassName.ToLower())
{
case ("cms.news"):
// handler for news item
break;
case ("cms.blogpost"):
//handler for blog post
//
// Form the URL used for shortening and then for the tweet
string docURL = "http://" + CMSContext.CurrentSite.DomainName + UrlHelper.ResolveUrl(DocumentURLProvider.GetUrl(newsDoc.NodeAliasPath, newsDoc.DocumentUrlPath));
//shorten the URL with bit.ly
string apiKey = "Your API Key";
string login = "Your Login";
string url = string.Format("http://api.bit.ly/v3/shorten?login={1}&apiKey={2}&uri={0}&format=txt",HttpUtility.UrlEncode(docURL), login, apiKey);
WebRequest req = WebRequest.Create(url);
WebResponse resp = req.GetResponse();
Stream stream =resp.GetResponseStream();
StreamReader sr = new StreamReader(stream);
String shortUrl =sr.ReadToEnd();
//Form the post text used for the tweet - remember can only be 140 characters
String postText = "Blog Post:" + newsDoc.DocumentName + "-" + shortUrl;
// Create the Yedda object
Yedda.Twitter t = new Yedda.Twitter();
t.Update("twitter username", "twitter password", postText, Yedda.Twitter.OutputFormatType.XML);
break;
default:
break;
}
}