Introduction to the Windows Communication Foundation
Services seem to be everywhere these days and even more ways to build them. Windows Communication Foundation (WCF) is the unified .NET programming model for building service oriented applications. It is designed to simplify the creation of connected applications through a service oriented programming model. WCF supports a variety of distributed application development by providing a layered architecture. The base of WCF provides the asynchronous message passing primitives. The higher level services layered on top of this base include secure and reliable messaging exchange, a typed programming model including serialization facilities, queued and transacted messaging exchange, integration with other programming models like MSMQ, COM+ and ASP.NET Web Services, Web Service Enhancements and others. Technically, WCF is implemented as a set of classes starting with the.NET Framework 3.0. The main namespace for working with WCF is the System.ServiceModel. This namespace contains the classes, enumerations and interfaces necessary to build WCF services and client applications.
A Windows Communication Foundation service is provided by defining one or more Windows Communication Foundation endpoints. An endpoint is defined by an address, a binding and a service contract. The address defines where the service is located. The binding specifies how to communicate with the service. The service contract defines the operations that the service can perform. WCF services must be hosted within a process to become active. The hosting environment is responsible to create and control the context and lifetime of the service. WCF services are designed to run in any Windows process that supports managed code. This programming model is designed to remain consistent and is considered independent of the runtime environment that is used to deploy the service. The benefit is that this makes the code for your service look very much the same whatever hosting option is selected.
Typically hosting options range from running inside a console or Windows Form application to server environments running within a worker process managed by IIS. WCF allows developers to choose the hosting environment that meet the needs of their application as opposed to requiring the use of a specific transport or technology.
In this article we will generate a simple WCF service using Visual Studio 2008 and the .NET Framework 3.5 and test it using the built in harnesses.
Creating the Service
1. Select New Project dialog Box, Click WCF and then select WCF Service Library, select Ok as shown below
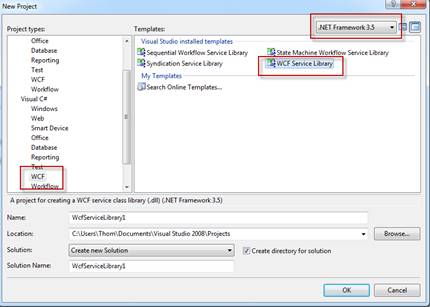
2. Press F5 to run the service and this will display the test client as shown below
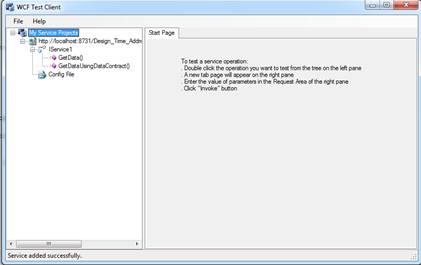
3. Double click the GetData Method under the IService1 to access the GetData tab displayed below
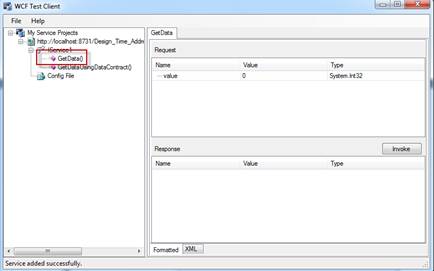
4. Select the value field enter 10 and click Invoke as shown below
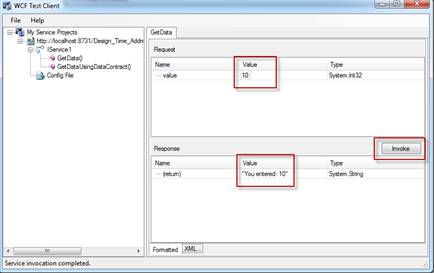
Note: if you receive a security warning select OK
Using the data contract
If you look at the code generated by the default service class you can see that there is a [ServiceContract] attribute and an [OperationContract] for each exposed operation. The design of WCF uses these attributes to expose the service endpoints and to determine the mapping of incoming and outgoing data between the worlds of .NET and SOAP. For example if you look at the generated code you see the following
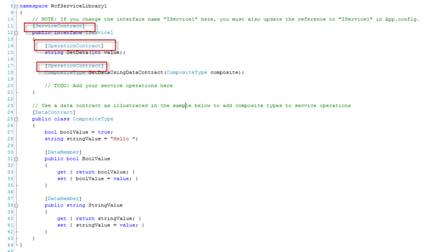
At run time WCF uses the service contract to perform the dispatching and serialization of data. The process of dispatching determines which methods are called for an incoming SOAP message. Serialization is the process of mapping between data found in the SOAP message and the corresponding .NET objects for the method call. By default, WCF uses a version of the XMLSerialize engine called the data control. By definition the data contract is a formal agreement between a service and a client that abstractly describes the data to be exchange. This means that in order to communicate the client and the service do not have to share the data types, only the same data contracts. This contract defines for each parameter or return type what the data is serialized or de-serialized for exchange. To keep the programming model simple, WCF includes built in data contracts for the .NET Framework primitive types; like integers and strings. Also several other types like DateTime and XMLElement. The default contract is defined by the types used in the method signature exposed by the [OperationContract]
Of course most applications contain complex or custom types for data interchange. Beyond the normal .NET Framework primitives WCF requires a customized data contract to influence the serialization process. This is done by applying the [DataContract] attribute to the classes, structure and enumerations. Once the target method has been determined, WCF relies on the methods data contract to perform the serialization. The [DataMember] attribute is then applied to each member of the data contract to indicate if it should be serialized.
To use the test harness for the generated data contract follows these steps:
1. Press F5 to start the application and within the test harness select GetDataUsingDataContract()
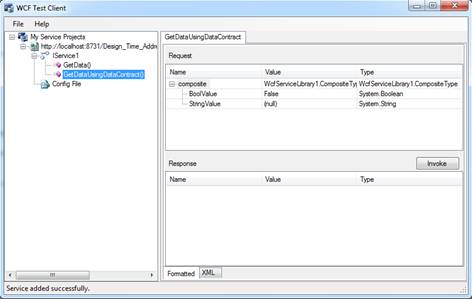
2. Enter the values shown below and select Invoke
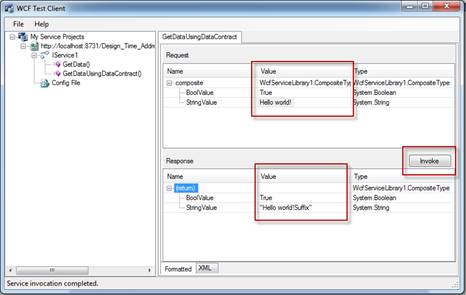
Thats the basics of using WCF with the built in test harness. There is an incredible amount of features available with WCF. In later posts I will build out some example services that can be consumed with an ASP.NET application like Kentico CMS.