Excluding content of Editable text from translation submissions
Translation submissions always contain the content entered into Editable text web parts. This occurs even though the web part property has the Translate field property set to false. In this article, we will discuss one approach for completely excluding this content from translation submissions.
This is a proof of concept which has been tested on Kentico 11 and 12
Current situation
Currently, when a page is submitted for translation any content entered into Editable text web part is included. Normally, you can exclude such content on Properties tab of the web part by selecting the corresponding field and setting Translate field to false. However, this doesn’t apply to Editable text web part by default. The reason for this is that the content of an editable region is always included in the page’s DocumentContent field.
Quick workaround
One way to get around this issue is to include instructions in the submission’s note to not translate the text. This is rather inconvenient as the note could be overlooked by translators or you may forget to add the note to some submissions.
Solution
A better way to deal with this is to scrap this note-leaving idea and exclude the content from the submission completely. Let me explain how to do that.
By creating a global event handler, you can remove the Editable text web part’s content completely from the submission. The global event handler will work alongside a custom table which will hold the IDs of the web part controls whose content is to be excluded.
So, in practice the workflow would be as follows:
- Place an Editable text web part on page and populate it with text
- Add its web part control ID to the custom table
- Submit the page for translation– at this point the content of the Editable text is removed
Let’s go through an example of the set up. In my case, I’ve created a custom table whose code name is customtable.WPToExclude. The table has a field with Field name of WPControlID and the Data type is “Text.”
That's all for our custom table! Now let’s take a look at the event handler’s code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Xml;
using CMS;
using CMS.CustomTables;
using CMS.DataEngine;
using CMS.DocumentEngine;
using CMS.TranslationServices;
// Registers the custom module into the system
[assembly: RegisterModule(typeof(WpToExcludeModule))]
public class WpToExcludeModule : Module
{
// Module class constructor, the system registers the module under the name "WpToExcludeModule"
public WpToExcludeModule()
: base("WpToExcludeModule")
{
}
// Contains initialization code that is executed when the application starts
protected override void OnInit()
{
base.OnInit();
// Assigns custom handlers to events
TranslationEvents.CreateSubmissionItem.After += Translation_CreateSubmission_After;
}
private void Translation_CreateSubmission_After(object sender, CreateSubmissionItemEventArgs e)
{
// Get WP IDs from the custom table
// Prepares the code name (class name) of the custom table
string customTableClassName = "customtable.WPToExclude";
// Gets the custom table
DataClassInfo customtable = DataClassInfoProvider.GetDataClassInfo(customTableClassName);
List<string> codeNames = CustomTableItemProvider.GetItems(customTableClassName)
.Column("WPControlID")
.Select(q => q["WPControlID"].ToString())
.ToList();
var xliff = e.SubmissionItem.SubmissionItemSourceXLIFF;
// Load XLIFF
XmlDocument doc = new XmlDocument();
doc.LoadXml(xliff);
// Select all Editable texts
XmlNodeList nodes = doc.SelectNodes("/xliff/file/body/trans-unit[starts-with(@id, 'editable')]");
foreach (XmlNode node in nodes)
{
// Check whether the Web part control ID is in the custom table
string[] splitID = node.Attributes["id"].Value.Split(';');
if (splitID.Length > 1 && codeNames.Contains(splitID[1]))
{
// Get the parent node
XmlNode parent = node.ParentNode;
// Remove the child node
parent.RemoveChild(node);
}
}
e.SubmissionItem.SubmissionItemSourceXLIFF = doc.OuterXml;
TranslationSubmissionItemInfoProvider.SetTranslationSubmissionItemInfo(e.SubmissionItem);
}
}
Now I just add the Editable text web part to a page. You can see its ID is “editabletext_excluded”.

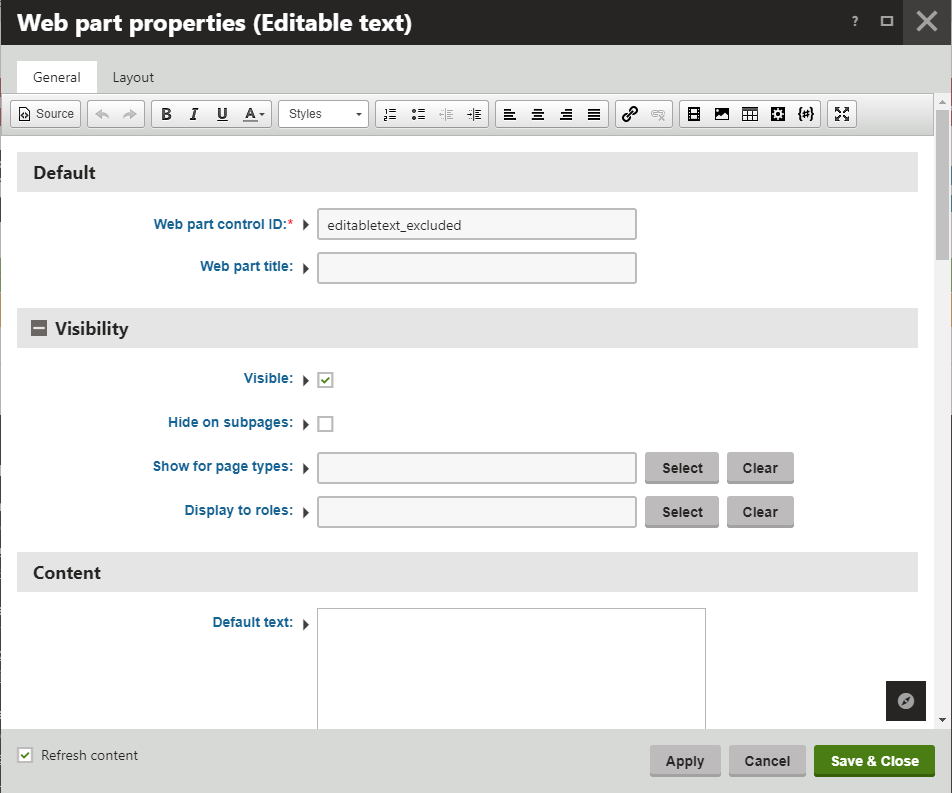
Next, I add the ID to the custom table.

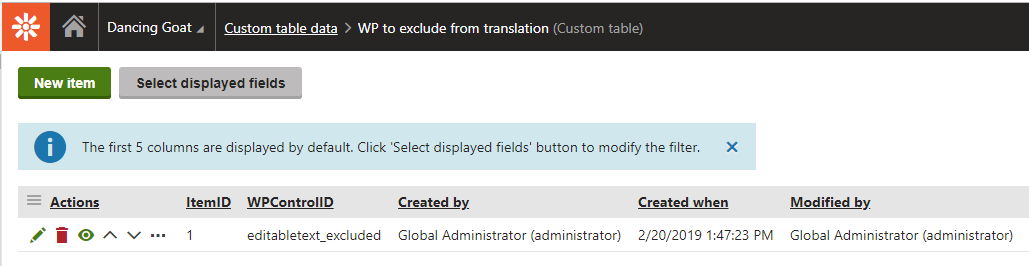
For demonstration, I’ve added another editable text web part. But, its ID is not present in the custom table which means that it won’t be excluded from the translation.

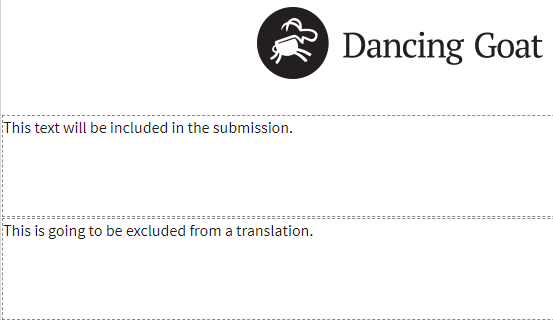
When I create a new culture version and submit the page for translation, the editable region is excluded from the submission.

