This chapter will guide you through the process of creating a very simple "Hello world" web part that displays a label and a button. When the button is clicked, it updates the current time displayed in the label.
1. | Open the web project in Visual Studio 2005 (or Visual Web Developer) using the WebProject.sln file or using File -> Open -> Web site in Visual Studio.
|
2. | Right-click the CMSWebParts folder in the Solution Explorer window and choose New Folder. Rename the folder to MyWebParts.
|
3. | Right-click the MyWebParts folder and choose Add New Item. Choose to create a new Web User Control and call it HelloWorld.ascx.
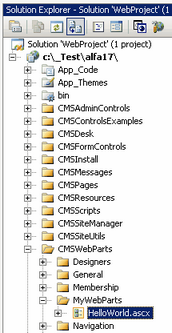
|
4. | Display the HelloWorld control on the Design tab. Drag and drop a new Button control and a new Label control on the form:
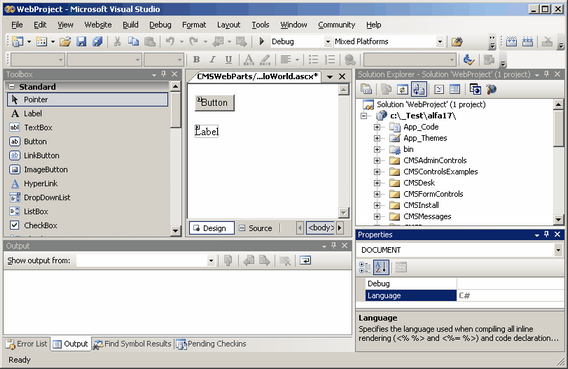
|
5. | Double-click the Button control and add the following code to the Button1_Click method:
|
[C#]
Label1.Text = DateTime.Now.ToString();
|
[VB.NET]
Label1.Text = DateTime.Now.ToString()
|
6. | Add the following line to the beginning of the code:
|
[C#]
using CMS.PortalControls;
|
[VB.NET]
Imports CMS.PortalControls
|
7. | Change the following line:
|
[C#]
public partial class CMSWebParts_MyWebParts_HelloWorld : System.Web.UI.UserControl
to
public partial class CMSWebParts_MyWebParts_HelloWorld : CMSAbstractWebPart
|
[VB.NET]
Partial class CMSWebParts_MyWebParts_HelloWorld
Inherits System.Web.UI.UserControl
to
Partial class CMSWebParts_MyWebParts_HelloWorld
Inherits CMSAbstractWebPart
|
It ensures that the user control behaves as a web part.
8. | Add the following code to the Page_Load method:
|
[C#]
Button1.Text = (string) PartInstance.GetValue("ButtonText");
|
[VB.NET]
(Visual Basic.NET doesn't create the Page_Load method automatically, so you need to add the whole method:)
protected Sub Page_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load
Button1.Text = CType(PartInstance.GetValue("ButtonText"), String)
End Sub
|
It sets the button text to the value configured in Kentico CMS Desk.
10. | Open Site Manager -> Development -> Web parts, click the root and click New category. Enter My web parts into the Category name field and click OK.
|
11. | Click the new category and click New web part. Choose to create a new web part and enter the following values:
- Web part display name: Hello world
- Web part code name: HelloWorld
- Web part file name: MyWebParts/HelloWorld.ascx
Click OK.
|
12. | Switch to the Properties tab and add the following property:
- Attribute name: ButtonText
- Attribute type: Text
- Attribute size: 100
- Field caption: Button text
- Field type: Text Box
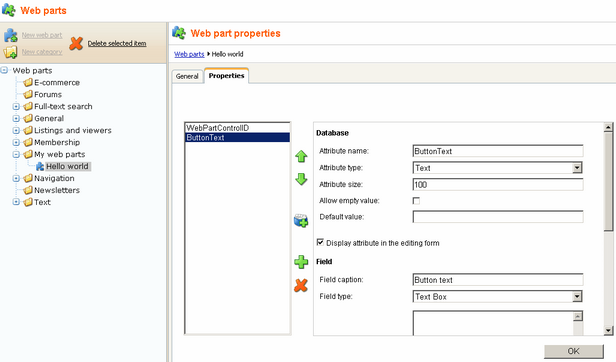
|
14. | Create a new blank page using the Simple layout (or any other layout) under the root and switch to the Design tab.
|
15. | Click Add web part in the upper right corner of the web part zone and choose to add the Hello world web part:

|
16. | The Web part properties window of the HelloWorld webpart appears, set the value of the Button text field to Hello world!
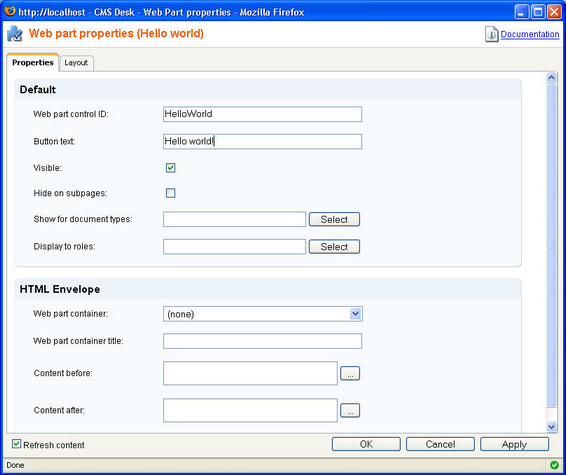
|
17. | Now switch to the Live site mode using the button in the main toolbar. You will see the button with text Hello world! When you click it, the label displays current date and time:

|
You have learned how to create a simple web part.

|
Tip: Displaying content on the web part
You can also use Kentico CMS Controls on the web part (on the ASCX control) to display content from Kentico CMS in a customized form.
|

|
Error message "The control collection cannot be modified during DataBind, Init, Load, PreRender or Unload phases."
If you get this error message you may need to modify the code of your web part, so that it doesn't display any content on the Design tab - for example:
[C#]
using CMS.PortalEngine;
public override void OnContentLoaded()
{
base.OnContentLoaded();
if ((this.PagePlaceholder.ViewMode == ViewModeEnum.Design)
|| (this .HideOnCurrentPage) || (!this.IsVisible))
{
this.Repeater1.DataSourceID = "";
this.CMSRepeater1.StopProcessing = true;
}
}
|
|

|
Initializing Kentico CMS controls in your custom web parts
If you are using Kentico CMS controls in your web parts, it is recommended to initialize the controls' properties using the combination of OnContentLoaded and SetupControl methods. This is the way it is handled in all Kentico CMS web parts. You can see the code of any of the web parts located in <project folder>/CMSWebParts and take it as an example.
If you are using classical .NET controls or third party controls, this can be handled in the PageLoad method.
If a problem occurs (e.g. on postback), try loading the control dynamically. This can be achieved by making a control from the web part and loading it dynamically (e.g. using the General -> User control web part).
|
|