How To: Split a String using System.String.Split
A fairly common task is parsing strings. One of the easiest ways that I found to accomplish this is to use the System.String.Split method. In this post we will look at a simple console application that uses this method. We will create an array of characters that are used to determine which characters are used as the delimiters in the returned array of string.
In this console application I start by building an array of delimiters and then pass them to the Split method and each word in the sentence is displayed using the resulting array of strings.
using System;
using System.Collections.Generic;
using System.Text;
namespace SplitExample
{
class Program
{
static void Main(string[] args)
{
//Build the list of delimeters
char[] delimiterChars = { ' ', ',', '.', ':', '\t' };
string text = "item1\titem2 item3:item4,item5 item6 item7";
System.Console.WriteLine("Input Text: '{0}'", text);
//Split them apart
string[] words = text.Split(delimiterChars);
System.Console.WriteLine("{0} Number words in text:", words.Length);
//display them out
foreach (string s in words)
{
System.Console.WriteLine(s);
}
Console.WriteLine("Press enter to continue");
Console.ReadLine();
}
}
}
When run it parses the strings as follows
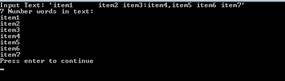