Display Bundled Items in your Shopping Cart Overview
This article shows you how to create a custom transformation that displays bundled items in your shopping cart overview and order summary pages.
Bundling products is a great sales vehicle for E-commerce sites, as it creates opportunities for bulk pricing, and allows customers to conveniently purchase similar items without having to add multiple products to their shopping cart. However, it can be easy for customers misread or forget what items are contained within various bundles. Below are instructions for creating a custom transformation that will display items contained within a bundle during the checkout process or while viewing the shopping cart.
How are bundles and products within the bundle related?
Bundles depend upon the COM_SKU and COM_Bundle tables. In the COM_SKU table, the bundle product has its own SKUID that is separate from the items contained within the bundle. This SKUID is the same as the BundleID in the COM_Bundle table for each of the items that are contained within the bundle.
For example, on the E-Commerce sample site there is a bundle product The Lord of the Rings: Trilogy Bundle (E-books) that has SKUID 115 and is a SkuProductType of BUNDLE. This bundle contains three items: The Lord of the Rings: The Fellowship of the Ring (E-book), The Lord of the Rings: The Return of the King (E-book), and The Lord of the Rings: The Two Towers (E-book).
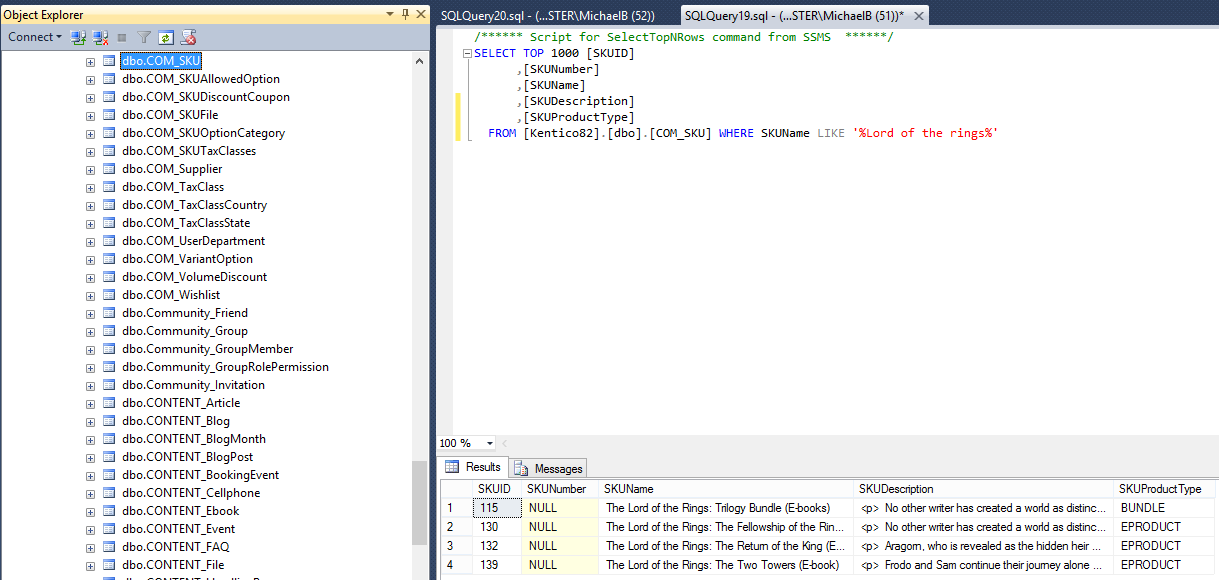
Each of these products within the bundle have their own unique SKUID, but also have the BundleID 115 in the COM_Bundle table, which is also the SKUID of the product bundle.
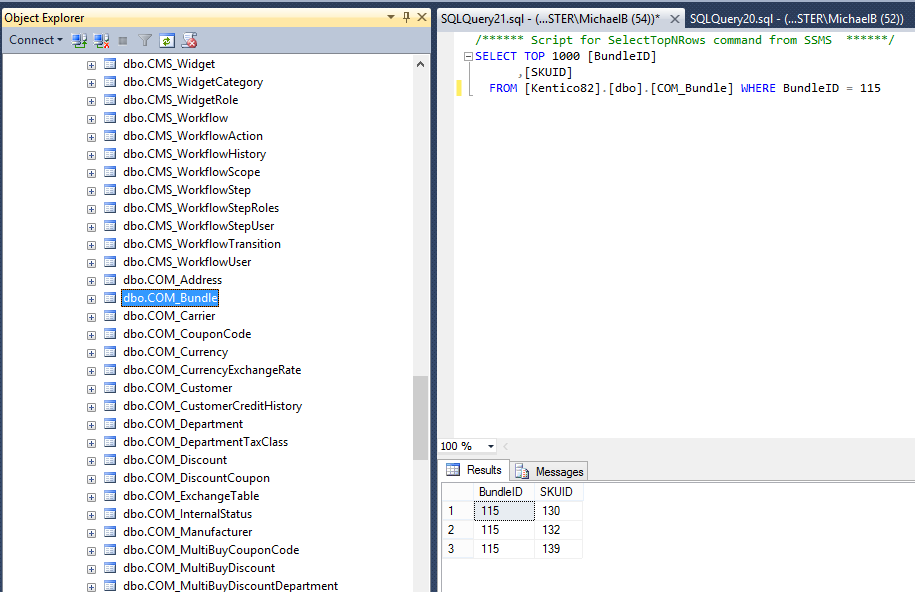
Now that we know how the bundle product and the items within it are related, we can create a custom transformation that uses a data query to join the COM_SKU and COM_Bundle tables and return each product within the bundle.
Code breakdown:
1. Pass the custom transformation SKUID and Product Type
<%# CustomBundleItems(Eval<int>("SKUID"), EvalText("SKU.SKUProductType")) %>
In this example, I have named my custom transformation CustomBundleItems. In order for the custom transformation to work appropriately, we need to pass the SKUID and Product Type of the products within your shopping cart to the custom transformation. This allows us to evaluate whether a product is a bundle and provides us the BundleID for the products within the bundle, since the bundle product’s SKUID is the same as the BundleID for the products contained.
2. Identify if the product is a bundle
Since this transformation is specific to bundles, we do not want to change how non-bundle products display in our shopping cart.
public string CustomBundleItems(int skuid, string productType)
{
//Ensure skuid is not null to avoid errors; only run for bundles
if (skuid != null && productType == "BUNDLE")
3. Once we have identified that the product’s SKUID is not null and that the product is a bundle, we use a data query to join the COM_SKU and COM_Bundle tables, and return all of the objects where the BundleID is equal to the skuid that we passed into the transformation.
//Object query that joins COM_SKU and COM_Bundle tables
//on SKUID and returns SKUInfo objects present in the current bundle
var currentBundle = SKUInfoProvider.GetSKUs().Source(sourceItem => sourceItem.Join<BundleInfo>("SKUID", "SKUID"))
.WhereEquals("BundleID", skuid);
4. After storing the SKUInfo objects within the bundle in a variable, we can then loop through the products and create a line broken list of the SKUInfo objects’ names into a simple string.
//initialize empty string to store SKUInfo items
string items = string.Empty;
//loop through the current bundle and set item SKUName property
foreach (SKUInfo item in currentBundle)
{
items += item.SKUName + "<br/>";
}
//Return the string of line broken items
return items;
Transformation code:
<%# CustomBundleItems(Eval<int>("SKUID"), EvalText("SKU.SKUProductType")) %>
Complete code behind:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using CMS.Ecommerce;
namespace CMS.Controls
{
public partial class CMSTransformation
{
//Pass SKUID for DataQuery and SKU.SkuProductType to evaluate if product is a bundle
public string CustomBundleItems(int skuid, string productType)
{
//Ensure values are not null to avoid errors
if (skuid != null && productType == "BUNDLE")
{
//Object query that joins COM_SKU and COM_Bundle tables
//on SKUID and returns SKUInfo items present in the current bundle
var currentBundle = SKUInfoProvider.GetSKUs().Source(sourceItem => sourceItem.Join<BundleInfo>("SKUID", "SKUID"))
.WhereEquals("BundleID", skuid);
//initialize empty string to store SKUInfo items
string items = string.Empty;
//loop through the current bundle and set item SKUName property
foreach (SKUInfo item in currentBundle)
{
items += item.SKUName + "<br/>";
}
//Return the string of line broken items
return items;
}
return null;
}
}
}
See also:
http://devnet.kentico.com/articles/kentico-8-technology-dataquery
http://devnet.kentico.com/articles/kentico-8-technology-dataquery-advanced-api
http://devnet.kentico.com/docs/8_2/api/html/R_Project_Kentico_API.htm